There are many reasons to build a content feed that will fit multiple screens. We see them in hotel ballrooms, at event spaces, co-working spaces, and more. These feeds can easily be built with HTML, CSS, and some vanilla Javascript. You can check out the final product in this Codepen:
See the Pen WeWork Elevator Screen Example by Randy Apuzzo (@ardeay) on CodePen.
Best Viewed in Split Screen at 0.5x or Click for Full Screen View
Of course, there are a lot of frameworks and options when working with HTML and Javascript. There are some amazing frameworks and tools like React, Vue, JQuery, Node, WebPack etc. etc., It can be both overwhelming and overkill to jump into a framework when accomplishing tasks. Javascript and HTML itself offers plenty to get you going.
In this tutorial, We are going to cover six awesome functionalities of Javascript:
QuerySelector: Selecting DOM elements with Query Selectors
Classlist: Using Class List to manage CSS classes
ForEach: Iterating over and manipulating an array of DOM elements
AddEventListener: Listen and act upon user interaction with event listeners
CreateElement: Create and insert a dynamic element into the DOM
Fetch: Getting External JSON Data with Fetch
As we explain each one, we are going to build on their functionality. In the end we are going to tie together each thing we learned to make a dynamic single page headless app.
Query Selectors
Javascript gives you many ways to select HTML DOM (Document Object Model) elements. List Items li, Divisions div, and Header One h1 are examples of DOM elements. Here are three common selectors:
Query selector lets you target one specific element, here is an example:
var myHeader = document.querySelector(‘h1’)
Which will return you the first h1 element found as a Node Object.
Query selector All is just like Query Selector, but it returns you all elements in an array, here is an example:
var myListItems = document.querySelectorAll(‘li’)
Which will return every li element found as an array of Node Object.
This is a nostalgic javascript method for me, as it was the primary way I would select an element growing up. This methods requires you to have an ID set on your element like <div id=”myDivision”></div>. Here is an example:
var myDiv = document.getElementById(‘myDivision’)
It returns a Node Object of the element you are targeting.
ClassList
What makes HTML magical is the ability to design any experience you want with CSS. Javascript lets you change the CSS realtime, and also apply CSS in the ready realtime. ClassList is a relatively new functionality that does not work well in Internet Explorer 10 and back. Let's take a look at an example and write some CSS to play with:
HTML
CSS
Javascript
That will make the background color of your header one h1
tag yellow by applying a class to your DOM Node! See a working example here:
See the Pen Query Selector Example by Randy Apuzzo (@ardeay) on CodePen.
ForEach
The forEach functionality in Javascript lets us iterate through an array of values and apply a function to each value. It’s a huge time saver and makes for clean code. Let’s look at an example:
HTML
CSS
JS
That code will make all list items have class yellowBackground applied to them. Very cool!
See the Pen Query Selector Example by Randy Apuzzo (@ardeay) on CodePen.
AddEventListener
Event listeners listen to user actions like mouse clicks or keystrokes. When an action is detected, you can run a function. Listeners are the foundation of interactive websites and web applications. Let’s see an example:
HTML
CSS
JS
This code gives each li a click listener. When the user click an element, the background will turn yellow!
See the Pen Query Selector Example by Randy Apuzzo (@ardeay) on CodePen.
You can listen to input changes, keystrokes, mousedowns, etc, be sure to check out this extensive list of things you can listen to.
Create Element
The ability to create new HTML realtime make for great dynamic experiences. Let’s have a look at Creating an Element and injecting into the dom realtime. Let’s look at an example:
HTML
CSS
JS
This new code sets up your click listener to add a new element on click, forever!
See the Pen Query Selector Example by Randy Apuzzo (@ardeay) on CodePen.
Fetch
My favorite part of Javascript is making requests to foreign servers and getting information back that can be presented to the user. Fetch is the latest way to do this in Javascript.
We are going to use Zesty.io headless content management system to provide a JSON endpoint of company listing. That can be setup for free, but we already create the content so you can see the JSON at this URL: https://meetupwework.zesty.dev/-/instant/6-faee919add-rp5gbb.json
Let’s see how we can access this information with a code example:
New HTML added to prior example:
New Javascript added to prior example:
This is a bit more code than before, but it’s doing more so that ok :). Notice we are using all of the functionality we learned above to make this happen.
See the Pen Query Selector Example by Randy Apuzzo (@ardeay) on CodePen.
Building the Single Page App
We are now going to make this all look like a real application using examples above, and some CSS magic. See the final code example here:
See the Pen WeWork Elevator Screen Example by Randy Apuzzo (@ardeay) on CodePen.
First presented at the Zesty.io meetupon October 14, 2019.
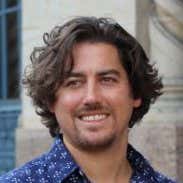
By Randy Apuzzo
Randy has had a penchant for computer programming from an early age and started applying his skills to build business software in 2004. Randy's stack of skills range from programming, system architecture, business know-how, to typographic design; which lends to a truly customer-centric and business effective software design. He leads the Zesty.io team as CEO.